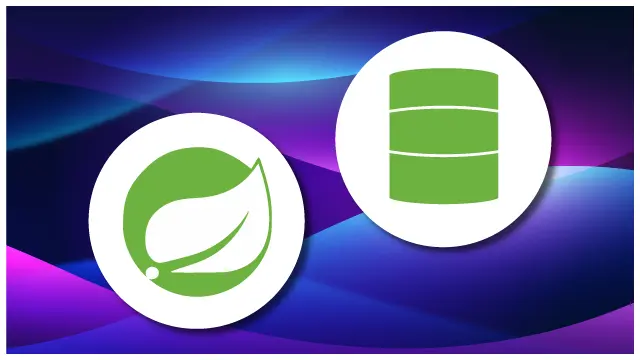
JPA | Learn Spring Boot, Spring Data JPA & Hibernate Basics
Spring Data JPA, Spring Boot with Rest API examples on PostgreSQL & also different databases like MySQL, MSSQL, MongoDB
Oak Academy
Summary
- Reed Courses Certificate of Completion - Free
Add to basket or enquire
Overview
Hi there,
Welcome to my "JPA | Learn Spring Boot, Spring Data JPA & Hibernate Basics" course.
Spring Data JPA, Spring Boot with Rest API examples on PostgreSQL & also different databases like MySQL, MSSQL, MongoDB
Spring Data JPA is a part of the larger Spring Data project, which aims to simplify data access and manipulation in Spring-based applications. Specifically, Spring Data JPA provides a high-level, abstracted approach to working with relational databases using the Java Persistence API
In the world of software development, simplification is often the key to productivity and efficiency. Spring Boot and Spring Data JPA are two powerful tools in the Spring Framework ecosystem that make it easier to develop robust and maintainable Java applications, especially when it comes to working with databases.
Spring Boot: The Foundation of Modern Java Applications
Spring Boot is a part of the Spring Framework that is designed to simplify the process of building production-ready applications. It achieves this by providing a set of conventions and tools to eliminate the need for extensive configuration, boilerplate code, and a steep learning curve .
Spring Boot embraces the concept of "opinionated defaults" . It comes with predefined configurations for common scenarios, such as database connections, web servers, and templating engines . This allows developers to get started quickly without having to make countless decisions .
One of the standout features of Spring Boot is its auto-configuration. It automatically configures components based on the dependencies you include in your project. If you're using Spring Data JPA, Spring Boot will configure your data source, entity manager, and transaction management with sensible defaults.
Spring Boot includes support for embedded servers like Tomcat, Jetty, and Undertow . You don't need to deploy your application to an external server; Spring Boot packages the server with your application, making it easy to run and deploy.
Spring Data JPA, a part of the Spring Data project, simplifies database access in Java applications, specifically when working with relational databases using the Java Persistence API (JPA) .
Curriculum
Course media
Description
Hi there,
Welcome to my "JPA | Learn Spring Boot, Spring Data JPA & Hibernate Basics" course.
Spring Data JPA, Spring Boot with Rest API examples on PostgreSQL & also different databases like MySQL, MSSQL, MongoDB
Spring Data JPA is a part of the larger Spring Data project, which aims to simplify data access and manipulation in Spring-based applications. Specifically, Spring Data JPA provides a high-level, abstracted approach to working with relational databases using the Java Persistence API
In the world of software development, simplification is often the key to productivity and efficiency. Spring Boot and Spring Data JPA are two powerful tools in the Spring Framework ecosystem that make it easier to develop robust and maintainable Java applications, especially when it comes to working with databases.
Spring Boot: The Foundation of Modern Java Applications
Spring Boot is a part of the Spring Framework that is designed to simplify the process of building production-ready applications. It achieves this by providing a set of conventions and tools to eliminate the need for extensive configuration, boilerplate code, and a steep learning curve .
Spring Boot embraces the concept of "opinionated defaults" . It comes with predefined configurations for common scenarios, such as database connections, web servers, and templating engines . This allows developers to get started quickly without having to make countless decisions .
One of the standout features of Spring Boot is its auto-configuration. It automatically configures components based on the dependencies you include in your project. If you're using Spring Data JPA, Spring Boot will configure your data source, entity manager, and transaction management with sensible defaults.
Spring Boot includes support for embedded servers like Tomcat, Jetty, and Undertow . You don't need to deploy your application to an external server; Spring Boot packages the server with your application, making it easy to run and deploy.
Spring Data JPA, a part of the Spring Data project, simplifies database access in Java applications, specifically when working with relational databases using the Java Persistence API (JPA) .
In Spring Data JPA with Rest API course you will Learn;
Installing java jdk and most useful IDEs like eclipse and intellij.
Spring Basics
Lombok
Postman,
Docker
DBeaver
Postgre Database
Spring Rest API
ORM,
JPA,
Hibernate
Spring JPA Annotations
Spring JPA Methods
Query methods and Finder methods
sorting and pagination
Relationships of Entities
Using JPA with different Databases.
Let's examine the benefits of Spring Data JPA
Repository Abstraction
Spring Data JPA introduces the concept of repositories. These are interfaces that define common database operations, such as saving, retrieving, and deleting entities. You can create custom repository interfaces to define your own data access methods, and Spring Data JPA generates the corresponding implementations.
Query Methods
One of the standout features of Spring Data JPA is the ability to define query methods by method naming conventions. It can derive queries from method names, which makes it easy to create custom queries without writing SQL or JPQL queries manually. For example, a method named findByLastName(String lastName) in a repository interface will automatically generate a query to retrieve entities by their last name .
Automatic CRUD Operations
Spring Data JPA provides automatic implementations for common CRUD operations. You can save, retrieve, update, and delete entities with minimal code, allowing you to focus on your application's business logic.
Pagination and Sorting
Implementing pagination and sorting for your queries is straightforward with Spring Data JPA. It abstracts the complexities of database-specific query syntax, making it easy to retrieve subsets of data.
Integration with Spring Framework
Spring Data JPA seamlessly integrates with the broader Spring ecosystem. It works well with Spring's dependency injection, transaction management, and security features, creating a cohesive development experience .
When you combine Spring Boot and Spring Data JPA, you get a powerful toolkit for building Java applications that interact with databases. Spring Boot provides a strong foundation for your application, with auto-configuration, embedded servers, and cloud-native support, while Spring Data JPA streamlines your data access, reducing the need for boilerplate code and manual SQL queries
So, this course will be a great chance for you to progress faster in the software world
We will start to our course with installing java jdk and most useful IDEs like eclipse and intellij. After that we will learn basic information about spring and Lombok to use in our training. Then we will examine some other tools or apps to use in our training like sts tool, Postman, Docker and postgre Database.
After that we will learn basics of spring API . Because this training is about JPA and I want to teach Data jpa on API pages.
Then we will start spring data JPA.
We will learn some programming concepts like ORM, JPA, Hibernate, Spring data jpa and we will examine differences between them.
After that we will start to JPA with rest API on postgre SQL. We will learn spring annotations that used in JPA like
Entity
Id
Table
Column
Generated vale
Unique constraint
Auto Identity, Sequence.
Also we will examine JPA repository and most useful methods od JPA repository like
Save
Find by
Save all
Find all
Delete
Delete by Id
Count and exist by Id.
We will continue with Query methods and Finder methods. After that we will continue with JPQL.
Then we will learn sorting and pagination, relationships between tables like
One to one
One to many
Many to one and many to many.
Also we will examine the search functionality, transaction management and unit testing.
We will make all operations with postgre SQL database. but also we will change our database with difference databases like mysql, mssql, mongo etc.
I think these are enough reasons to start the course. If you're ready, let's get started.
Why would you want to take this course?
Our answer is simple: The quality of teaching.
When you enroll, you will feel the OAK Academy`s seasoned developers' expertise.
Step-by-Step Way, Simple and Easy With Exercises
Fresh Content
It’s no secret how technology is advancing at a rapid rate. New tools are released every day, Vue updates its system, and it’s crucial to stay on top of the latest knowledge. With this course, you will always have a chance to follow the latest trends .
Video and Audio Production Quality
All our content is created/produced as high-quality video/audio to provide you with the best learning experience .
You will be,
Seeing clearly
Hearing clearly
Moving through the course without distractions
You'll also get:
Lifetime Access to The Course
Fast & Friendly Support in the Q&A section
Dive in now!
We offer full support, answering any questions.
Dive in now into; "JPA | Learn Spring Boot, Spring Data JPA & Hibernate Basics" course.
Spring Data JPA, Spring Boot with Rest API examples on PostgreSQL & also different databases like MySQL, MSSQL, MongoDB
We offer full support, answering any questions.
See you on the other side!
Questions and answers
Currently there are no Q&As for this course. Be the first to ask a question.
Certificates
Reed Courses Certificate of Completion
Digital certificate - Included
Will be downloadable when all lectures have been completed.
Reviews
Currently there are no reviews for this course. Be the first to leave a review.
Legal information
This course is advertised on reed.co.uk by the Course Provider, whose terms and conditions apply. Purchases are made directly from the Course Provider, and as such, content and materials are supplied by the Course Provider directly. Reed is acting as agent and not reseller in relation to this course. Reed's only responsibility is to facilitate your payment for the course. It is your responsibility to review and agree to the Course Provider's terms and conditions and satisfy yourself as to the suitability of the course you intend to purchase. Reed will not have any responsibility for the content of the course and/or associated materials.